When creating forms, we can easily add placeholder text to input fields by setting the placeholder
attribute and value on an input
element like this: <input placeholder="Name" />
However, there is no such attribute for a select
element, also known as a drop-down or menu, so how can we mimic that feature? Specifically, how can we mimic that feature without sacrificing performance by adding JavaScript or jQuery? Let’s take a look.
Select Field Placeholder Tutorial
Time needed: 1 minute
Here is how to add a placeholder to a select field using only HTML and CSS.
- Set the required attribute on the select element.
This method works by targeting an invalid
option
. In order for anyoption
to be considered invalid, theselect
element must first be required. E.g.<select required></select>
- Create the placeholder as an option
The placeholder
option
needs to exist, e.g.<option>Please select your favorite fruit</option>
- Make the placeholder option invalid by giving it an empty value
When an
option
has an empty value for a requiredselect
element, the browser identifies it as invalid. Simply omitting the value is not enough, because the value is defaulted to the text. The empty value needs to be explicitly set, e.g.,<option value="">Please select your favorite fruit</option>
- Style the invalid option with CSS
Now that the invalid
option
exists for a requiredselect
field, it can be targeted and styled using the:invalid
CSS pseudo-class. E.g.select:invalid { color: #999999; }
Setting the disabled
attribute on the invalid option
element prevents the user from selecting it, and setting the hidden
attribute hides it completely. Setting the selected
attribute ensures it is displayed as the placeholder, even when it is disabled
and/or hidden
. Below is the code put all together.
HTML
<label>
<select required>
<option value="" selected disabled hidden>Please select your favorite fruit</option>
<option>Apple</option>
<option>Banana</option>
</select>
</label>
CSS
select {
color: #000000;
}
select:invalid {
color: #999999;
}
Contact Form 7 Integration
If you have a WordPress website with Contact Form 7 (CF7), this method can still be used. Unfortunately, it is not available out-of-the-box for Contact Form 7, but it is available in the free CF7 addon called Contact Form 7 – Dynamic Text Extension (DTX).
You can use the placeholder
attribute in any DTX form tag or use the form tag generator (learn more about that here). For most form tags, it’ll say “Dynamic placeholder” but for dynamic drop-down menu, it is called “First Option Label” (learn more about the dynamic select here).
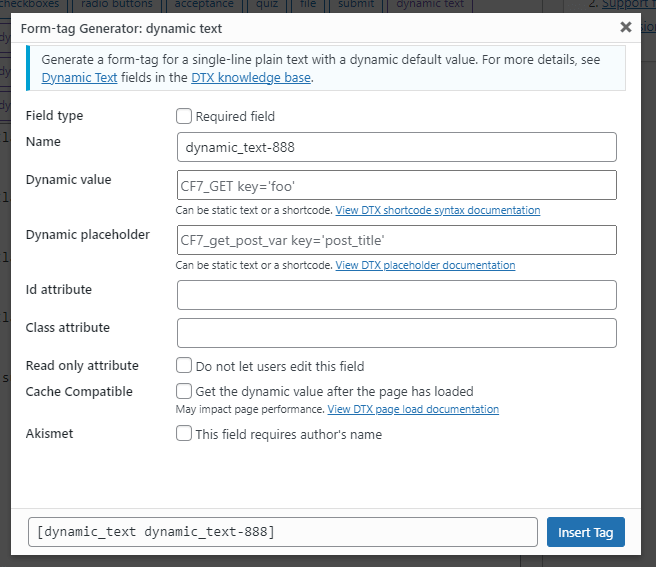
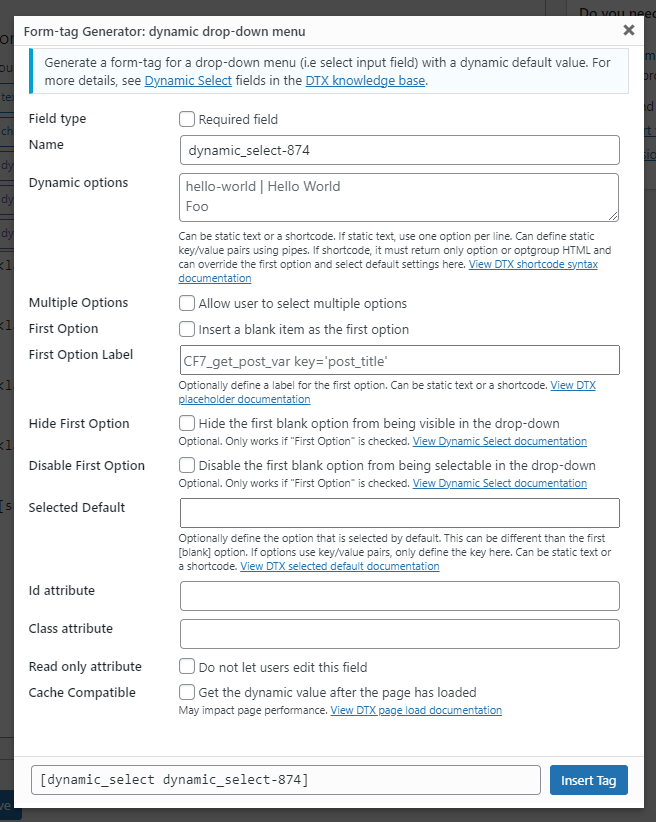
jQuery Validate Integration
Now this method is awesome, but it does have a drawback: the select field is required. This is only a drawback if you don’t want the select field to actually be required. If you’re using jQuery validation for frontend form validation, this is actually an easy fix.
- Keep the
required
attribute on theselect
element so the invalidoption
and CSS works - In the HTML, add
ignore
(or whatever custom class you want to use) to yourselect
element’sclass
attribute - In the JavaScript, set the
ignore
property to theignore
class (or whatever class you used) in yourvalidate
call (be sure to use CSS selector notation by prefixing it with a period).
See the coding example below.
HTML
<form id="my-form">
<label>
<select class="ignore" required>
<option value="" selected disabled hidden>Please select your favorite fruit</option>
<option>Apple</option>
<option>Banana</option>
</select>
</label>
</form>
JavaScript
jQuery('#my-form').validate({
'ignore': '.ignore'
});
Additional Resources
- My answer to the question, “Modify Select So Only The First One Is Gray” on Stack Overflow.
- My CodePen demo that explores additional select box styles using Font Awesome and shows this one in action on a light background.